Mastering Cursor: Features, Tips, and Best Practices
As a developer deeply invested in optimizing my workflow, I've spent significant time exploring and improving my use of Cursor. This comprehensive guide covers my mind dump of everything from basic setup to advanced features, helping you maximize your productivity with this powerful tool.
AI isn't just another dev tool - it's a fundamental shift in how we write code. The developers who master it aren't just more productive, they're redefining what's possible.
What is Cursor?
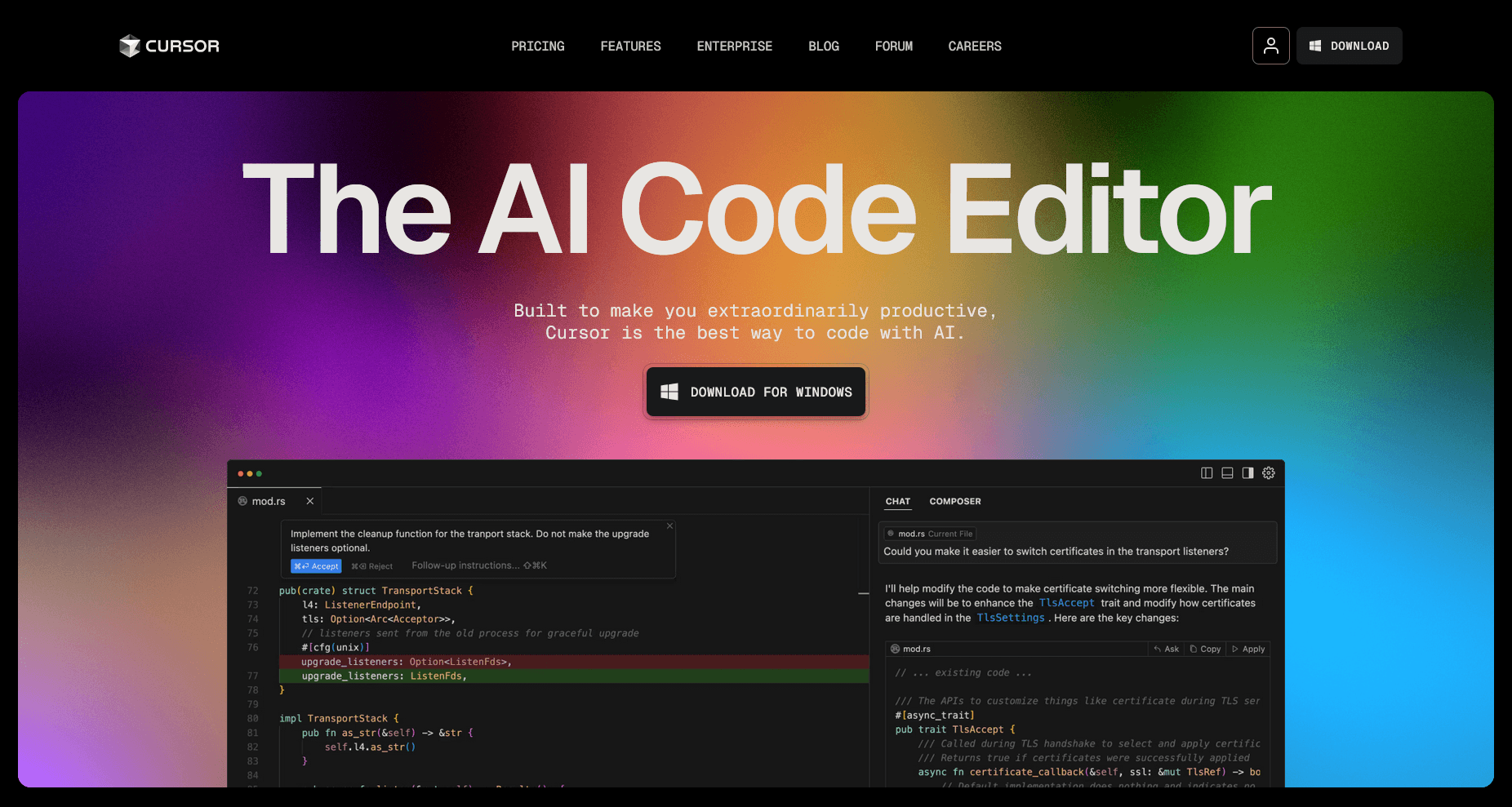
Cursor is a powerful AI-powered development IDE, built on top of VSCode, that can help you write code faster and more efficiently. It uses AI to understand your code and suggest changes, as well as to generate code based on your instructions.
Define your rules
Rules are a way to guide your chosen LLM to behave by providing more context.
At the heart of AI development is context.
- Not getting useful output? You need to provide more context.
- Not following your coding style? You need to provide more context.
- Not abiding by your naming conventions? You need to provide more context.
Everything is all about context.
Global rules
Global rules are applied directly to the IDE and will be used by all LLMs in all projects.
This provides a great place to define how you want your responses to look, feel, and behave.
You can edit this by going to File > Preferences > Cursor settings > Rules for AI
.
I'm using the same rules as the Cursor development team:
DO NOT GIVE ME HIGH LEVEL ****, IF I ASK FOR FIX OR EXPLANATION, I WANT ACTUAL CODE OR EXPLANATION!!! I DON'T WANT "Here's how you can blablabla"
- Be casual unless otherwise specified
- Be terse
- Suggest solutions that I didn't think about—anticipate my needs
- Treat me as an expert
- Be accurate and thorough
- Give the answer immediately. Provide detailed explanations and restate my query in your own words if necessary after giving the answer
- Value good arguments over authorities, the source is irrelevant
- Consider new technologies and contrarian ideas, not just the conventional wisdom
- You may use high levels of speculation or prediction, just flag it for me
- No moral lectures
- Discuss safety only when it's crucial and non-obvious
- If your content policy is an issue, provide the closest acceptable response and explain the content policy issue afterward
- Cite sources whenever possible at the end, not inline
- No need to mention your knowledge cutoff
- No need to disclose you're an AI
- Please respect my prettier preferences when you provide code
- Split into multiple responses if one response isn't enough to answer the question
- If I ask for adjustments to code I have provided you, do not repeat all of my code unnecessarily. Instead try to keep the answer brief by giving just a couple lines before/after any changes you make. Multiple code blocks are ok
Project specific rules
Project rules only apply to the project you've added them to.
This provides a great place to add context specific to the project you're working on.
You can add project specific rules by adding a .cursorrules
file to the root of your project.
Find framework specific rulesheets on cursor.directory/rules to use as a base and add your own modifications as needed.
Common modifications I usually include are:
# Project Name: [ name ]
## Project Description
[ description ]
## Context Initialization
Starting point for each interaction.
- ALWAYS read `.notes/project_overview.md` and `.notes/task_list.md`
## Project Structure, Architecture and Priorities
[ add details about your application architecture you want it to adhere to ]
Here is my product structure with priority weights as a decimal next to each folder.
[ tip: run a tree command on your project and paste it in here ]
eg:
├── .notes p: 1.0
│ ├── todo.md p: 1.0
│ ├── project_overview.md p: 1.0
│ ├── task_list.md p: 1.1
│ ├── directory_structure.md p: 1.3
│ └── meeting_notes.md p: 1.4
## State Management
[ tooling used for state mgmt ]
## Data Flow Patterns
[ how does data flow in your application? ]
## Preferred tooling
[ any component libs you want it to know about? what ORM? etc... ]
## Code Style and Formatting
[ any code style preferences you want it to know about? ]
## Quality Gates
[ any quality gates you want to maintain? ]
## Common Tasks
[ any common tasks that your routinely perform working on your project? ]
## Key Considerations
- Focus on core features
- Prioritize core features like user authentication, XXX, and YYY for the MVP
- Defer non-essential features to later iterations
- Prioritize maintainability
- Use meaningful variable and function names
- Add comments to explain why behind the code in more complex functions
- Keep functions small and focused (single responsibility)
[ ...add any other key considerations here ]
[ ...add one of the framework specific rulesheets here ]
This ensures both you and the AI are on the same page regarding priorities, coding standards, and how to approach tasks.
Remember you can choose to structure your .cursorrules
file however you want.
Pro Tip: You can use AI to help your create your .cursorrules
file.
Understand the modes
Cursor offers different modes that are useful for different tasks. Use them accordingly.
Chat Mode
This is useful for quick debugging, code reviews, and general questions.
Think of it as your AI pair programmer - great for:
- Quick code explanations
- Debugging help
- Best practice advice
- Code reviews
- Documentation help
Composer Mode ⭐
This is useful for new feature development and generally where I spend most of my time.
It comes in two flavors:
- Normal Mode
- Write code with AI assistance
- Get intelligent autocomplete
- Refactor existing code
- Generate new components/functions
- Agent Mode
- Handles complex, multi-step tasks
- Breaks down large features into manageable chunks
- Maintains context across multiple files
- Great for major refactoring
Agent Mode is still experimental. Always review its changes carefully.
Bug Finder
An AI-powered static analysis tool that:
- Scans your codebase for potential issues
- Identifies security vulnerabilities
- Spots performance bottlenecks
- Suggests fixes for common problems
- Works across multiple languages
Use Bug Finder before commits or PRs for an extra layer of code review.
YOLO Mode
Enable this in Settings > Features > "Enable YOLO Mode"
.
This feature allows Cursor agents to make autonomous edits:
- Auto-accept certain suggestions based on your configuration
- Make autonomous edits
- Work faster with less interruption
Only use YOLO mode on code with good version control. It can make unexpected changes.
Auto Context (Beta)
Found in Settings > Features > "Enable Auto Context Beta"
.
This feature is useful for adding additional context:
- Automatically detects relevant files
- Maintains context without manual @ references
- Learns from your coding patterns
- Reduces the need for manual context setting
Let it read your mind
When writing prompts, first write out your thoughts in a markdown file.
This will allow the autocomplete feature to work offering suggestions as you type.
Once you're happy with the prompt you can copy it out into the mode of your choice.
Use a CHANGELOG.md
Add a CHANGELOG.md
file to your project and update your .cursorrules
to include:
- ALWAYS read the changelog to refresh your memory on the changes made
- ALWAYS update the changelog as you make signficant changes
This will provide you with a record of the changes the agent has made as it works.
It serves multiple purposes as it provides the agant with a form of short/longterm memeory as you work on your project over time.
Learn the @ features
The @
symbol is your key to providing context to Cursor.
It offers a range of different tools to include content, it's worth familiarising yourself with them.
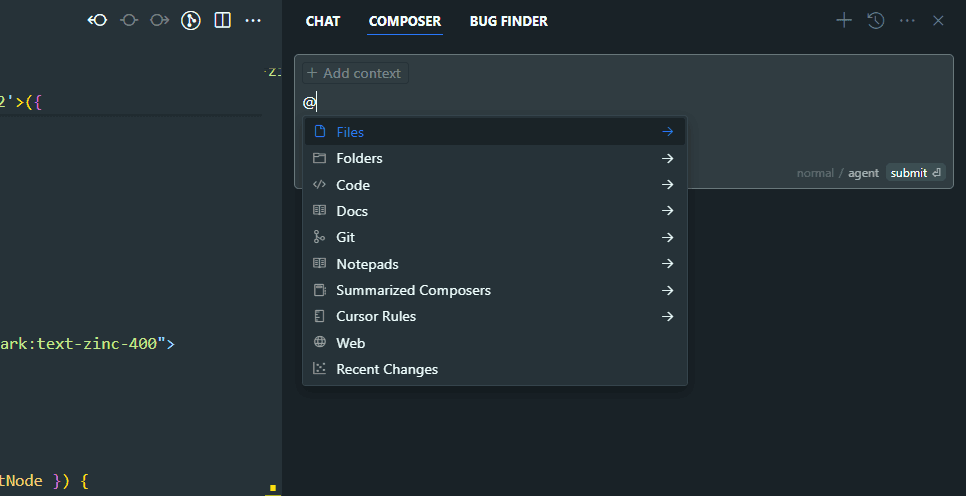
@Files
The most basic and frequently used context provider. Reference any file in your project to give Cursor access to its contents. Perfect for when you need to discuss specific implementations or make targeted changes.
Usage Example: @src/components/Button.tsx help me optimize this component
@Folders
View and reference entire directories at once. This is invaluable when you need to understand or modify related files as a group, or when implementing features that span multiple files in a directory.
Usage Example: @src/components/ list all components that use useEffect
@Code
Direct code snippets for discussion or modification. Unlike @Files, this feature is more focused on the code itself rather than its location in the project. Great for exploring implementations or debugging specific code blocks.
Usage Example: @Code help me understand this algorithm
@Docs
Access project documentation, README files, and other documentation resources. Essential for maintaining context about project architecture, requirements, and design decisions.
Usage Example: @docs/architecture.md how does this feature align with our current architecture?
@Git
Interface with your version control history and changes. Useful for understanding code evolution, reviewing changes, or discussing implementation history.
Usage Example: @Git what were the recent changes to the auth system?
@Notepads
Create and maintain temporary notes or scratchpads during your development session. Perfect for brainstorming, planning complex features, or keeping track of ideas.
Usage Example: @Notepads create a new note for refactoring ideas
@Summarized Composers
Access and reference previous conversations and coding sessions. This helps maintain context across multiple development sessions and ensures consistency in implementations.
Usage Example: @Summarized Composers what did we decide about the state management approach?
@Cursor Rules
Reference and modify your project's .cursorrules
file. This feature helps maintain consistent AI behavior and project-specific guidelines throughout your development process.
Usage Example: @Cursor Rules update typescript standards
@Web
Access external web resources and documentation without leaving your IDE. Useful for checking official docs, best practices, or external references.
Usage Example: @Web react hooks best practices
@Recent Changes
Track and review recent modifications to your codebase. Essential for maintaining awareness of project evolution and ensuring changes align with project goals.
Usage Example: @Recent Changes show me today's modifications to the API endpoints
Pro Tip: Combine multiple @
features to create rich context for your
queries.
Pro Tip: Instructing AI with this is my @backend
, this is my @frontend
,
now go do X is much more effective than just @codebase
, go do X.
Leverage the / feature ⭐
Getting tired of using @
features to reference files?
Use the /
feature to instantly reference active or open editors.
This feature is instantly powerful. It allows you to perform a manual search of your project and reference the results you want to target easily.
For example, imagine searching for all usages of a specific type or function, clicking through the results to select and open the ones you want to target and easily reference them in your prompt with /reference open editors
.
Leverage PRDs
Shout out to @traskjd for teaching me this one!
Product Requirements Documents (PRDs) are a great way to provide context to the AI.
These documents are battle tested methods of communicating requirements between stakeholders and developers. So why don't we use them to communicate with AI?
What's more, you can use AI to help you create your PRDs. To get started you could use this prompt:
I'd like you to help me create a Product Requirements Document (PRD) for the following use case:
Name: [application name]
Type: [application type]
Technology:
- [technology 1]
- [technology 2]
- [technology 3]
Capabilities:
- [capability 1]
- [capability 2]
- [capability 3]
User Stories:
- [user story 1]
- [user story 2]
- [user story 3]
Please as any questions to help build this final PRD, put it in markdown format.
After you answer any questions, you should be left with a PRD in markdown format. Create and add this to a .notes/prd.md
file in your project.
You can now leverage the PRD as a context file to help with your development using the agent to help implement the PRD for you.
Usage Example: using this @prd.md file, implement the new feature in @app
Working with bleeding edge frameworks ⭐
If you're working with a bleeding edge framework, you can use the @Web
feature to get the latest documentation and best practices.
You can then use Cursor to ingest the docs and come up with advice, which you then put in your .cursorrules
file.
Usage Example: @Web ingest the latest documentation for the latest version (16.0.1) of Next.js and put it in @Cursor Rules
Conclusion
Cursor is a powerful tool, the first I've used of it's kind. But it has changed the entire way I code. The key is to maintain a balance between leveraging its AI capabilities and preserving your development skills.
- Keep comprehensive documentation
- Use
.cursorrules
effectively - Refresh yourself on the latest features often
- Maintain good development practices
- Regularly review and update your workflow
The most important thing is to find a workflow that works for you while maintaining code quality and your development skills.
If you've found this guide helpful, or have any tips of your own, I'd love to hear from you. I'm always learning and exploring new ways to improve my development workflow, you can reach me on X or BlueSky.